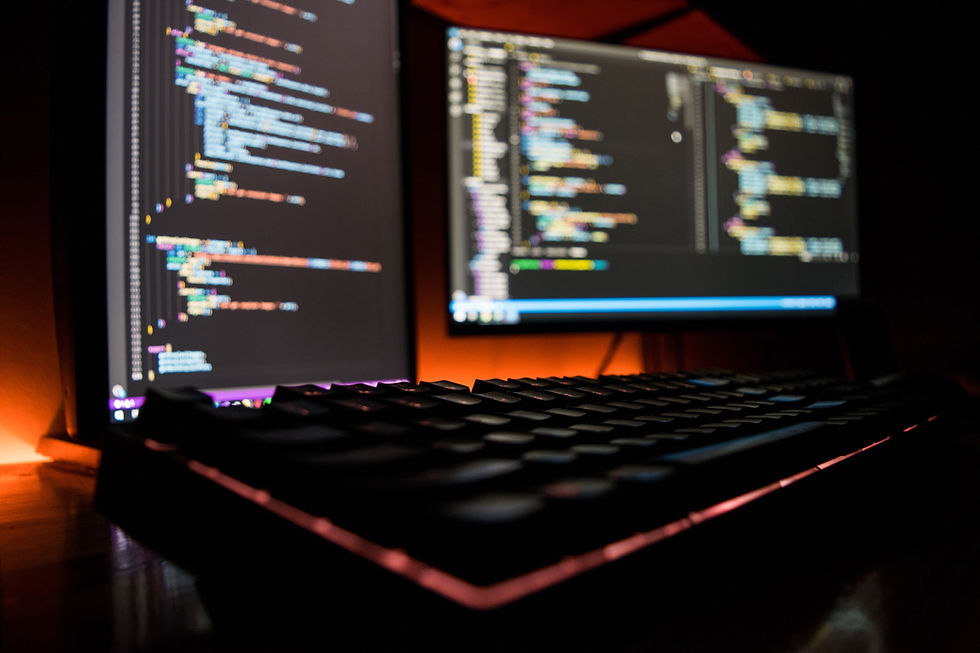
Python decorators – the hidden gems of Python programming that can take your code from mundane to magnificent with just a few lines of code. If you've been on a quest to enhance your Python programming skills, then mastering decorators is an essential step in your journey. Let's dive into the world of decorators and unravel the magic they bring to your Python code.
The Magic Behind Python Decorators
At their core, decorators are functions that modify the behavior of another function or method. They allow you to add functionality to your existing code without modifying the original function itself. This powerful feature enables you to encapsulate common functionalities, such as logging, authentication, or performance monitoring, and apply them across multiple functions effortlessly.
Unpacking the Syntax of Decorators
To define a decorator in Python, you simply prefix a function with the @decorator_name syntax before the function definition. This elegant syntax enhances readability and makes it clear that a decorator is being applied. By incorporating decorators, you can keep your code DRY (Don't Repeat Yourself) and maintain a clean, concise codebase.
Elevating Your Code with Decorator Use Cases
Imagine effortlessly logging the input parameters and output of a function with a simple decorator. Decorators empower you to add this functionality without cluttering your core codebase.
With decorators, you can effortlessly measure the execution time of your functions, gaining valuable insights into performance optimization opportunities.
Secure your application by implementing authentication logic through decorators. Control access to specific functions based on user authentication easily.
A Practical Example of Python Decorators
import time
import functools
# Step 1: Define the decorator
def execution_time_logger(func):
@functools.wraps(func)
def wrapper(*args, **kwargs):
start_time = time.time()
result = func(*args, **kwargs)
end_time = time.time()
execution_time = end_time - start_time
print(f"Function '{func.__name__}' executed in {execution_time:.4f} seconds")
return result
return wrapper
# Step 2: Apply the decorator to some functions
@execution_time_logger
def slow_function():
time.sleep(2)
return "Function complete"
@execution_time_logger
def fast_function():
return "Quick function complete"
# Test the decorated functions
print(slow_function())
print(fast_function())
Taking Your Python Skills to the Next Level
Mastering Python decorators can significantly elevate your code quality and maintainability. Embrace the power of decorators to streamline your code, enhance reusability, and encapsulate common functionalities efficiently.
In conclusion, Python decorators are a game-changer in the world of Python programming. By incorporating decorators into your projects, you can write cleaner, more elegant, and maintainable code. So, dive deep into decorators, explore their endless possibilities, and witness your Python code flourish like never before.
Now, armed with this newfound knowledge, unleash the true potential of Python decorators and witness the transformation they bring to your codebase!
By delving into the realm of Python decorators, you unlock a realm of possibilities for enhancing your code – from simplifying complex logic to adding reusable functionalities. Embrace the elegance of decorators and transform your Python code into a masterpiece of efficiency and elegance!
Comments